Python is known for its simplicity and readability, making it an ideal choice for both beginners and experienced programmers. Whether you're new to programming or looking to expand your skill set, Python is a great language to learn. In this article, we'll cover the basics to get you started.
What is Python?
Python is a high-level, interpreted programming language known for its clean and readable code. Its design philosophy emphasizes code readability and allows developers to express concepts in fewer lines of code than in languages like C++ or Java. Python is versatile and can be used for a wide range of applications, from web development to data analysis and scientific computing.
Installing Python
Before you can start writing Python code, you'll need to install it on your computer. Fortunately, Python is available for all major operating systems, including Windows, macOS, and Linux. To make the installation process easy for you, we'll guide you through the steps for each platform. Once Python is up and running, you're ready to dive into the world of programming.
Your First Python Program
Now that you have Python installed, it's time to write your first Python program. We'll begin with the classic "Hello, World!" example. This simple program will introduce you to the basic structure of Python code and get you comfortable with the Python interpreter. By the end of this section, you'll have written and executed your first Python script.
Python Fundamentals
With the basics covered, it's time to explore Python's fundamentals. These are the building blocks of Python programming, and understanding them is crucial as you progress in your journey.
Variables and Data Types
In Python, variables are used to store and manage data. We'll show you how to declare variables and work with different data types, including integers, strings, and lists. Understanding data types is essential for writing effective and efficient code.
Conditional Statements
Python allows you to make decisions in your code using conditional statements like if, elif, and else. You'll learn how to control the flow of your program and execute specific code blocks based on certain conditions. This is fundamental for creating dynamic and responsive applications.
Loops
Loops are essential for automating repetitive tasks. Python provides for and while loops, and we'll explore both types. You'll discover how to iterate through lists, perform calculations, and create efficient, repeatable processes in your code.
Functions and Modules
Python's modularity is one of its strengths. In this section, we'll delve into functions and modules, which allow you to organize your code effectively and extend your program's capabilities.
Functions
Functions are reusable blocks of code that you can define and call as needed. We'll show you how to create functions, pass parameters, and handle return values. This approach helps keep your code organized and makes it easier to maintain and debug.
Modules
Python's Standard Library and external modules offer a vast array of pre-written code for you to use. We'll introduce you to the Python Standard Library and show you how to import and utilize external modules. This will save you time and effort by harnessing the power of pre-built functionality.
Data Structures
Python provides a rich variety of data structures to suit different needs. Understanding how to work with these data structures is crucial for managing and organizing your data effectively.
Lists
Lists are versatile and fundamental data structures used to store and manipulate collections of data. We'll teach you how to create, modify, and access elements within lists, making them an invaluable tool for managing data in your programs.
Dictionaries
Dictionaries are Python's key-value pairs, and they have various practical use cases. We'll guide you through how to create and manipulate dictionaries, which are essential for tasks like creating configuration settings or managing data in a structured way.
Tuples and Sets
Tuples are used for immutable sequences, and sets store unique, unordered data. Understanding these data structures is essential for specific tasks, such as ensuring data integrity and uniqueness in your applications. We'll explore when and how to use them effectively.
Object-Oriented Programming (OOP)
Python supports object-oriented programming principles, which help you build complex, organized code. In this section, we'll introduce you to the concepts of classes, objects, inheritance, and polymorphism.
Classes and Objects
Classes are used to model real-world concepts, and objects are instances of these classes. You'll learn how to define classes, create objects, and understand the concept of attributes and methods within them.
Inheritance and Polymorphism
Inheritance allows you to create new classes based on existing ones, promoting code reuse. Polymorphism enables you to work with objects in a more flexible and generic way. We'll explore how these concepts can improve the structure and organization of your code.
Error Handling and Debugging
Even experienced developers make mistakes, and Python provides tools to deal with errors effectively. In this section, we'll discuss exception handling and debugging techniques.
Exception Handling
Discover how to handle exceptions gracefully using try, except, and finally blocks. Exception handling allows your program to recover from errors or respond appropriately, enhancing the robustness of your code.
Debugging Techniques
Learn how to identify and fix errors using Python's debugging tools and strategies. Debugging is a critical skill in any programmer's toolbox, and Python provides various methods to help you locate and resolve issues in your code.
Working with Files and Data
Python can handle various types of data, including files and databases. In this section, we'll explore file handling and manipulating structured data using libraries for JSON and CSV.
File Handling
Reading and writing data to files is a common task in programming. We'll show you how to open, read, write, and close files, and explain the different file modes. You'll be able to manage and store data in files efficiently.
Working with JSON and CSV
Structured data is prevalent in many applications. We'll introduce you to Python's built-in libraries for working with JSON and CSV files, enabling you to manipulate data in formats commonly used for configuration, storage, and data exchange.
Introduction to Python Libraries
Python's extensive library ecosystem enhances its capabilities. In this section, we'll introduce you to some essential libraries for data analysis, data visualization, and web scraping.
NumPy and Pandas
NumPy and Pandas are powerful libraries for data analysis and manipulation. You'll explore how these tools can help you work with large datasets, perform calculations, and gain insights from your data.
Matplotlib and Seaborn
Data visualization is a crucial aspect of data analysis. Matplotlib and Seaborn are popular libraries for creating stunning data visualizations. We'll provide an overview of how to use them to present data effectively.
Requests and BeautifulSoup
Web scraping is the process of extracting data from websites. We'll get you started with Requests, a library for making web requests, and BeautifulSoup, a library for parsing HTML and XML. You'll learn how to gather data from websites for various purposes.
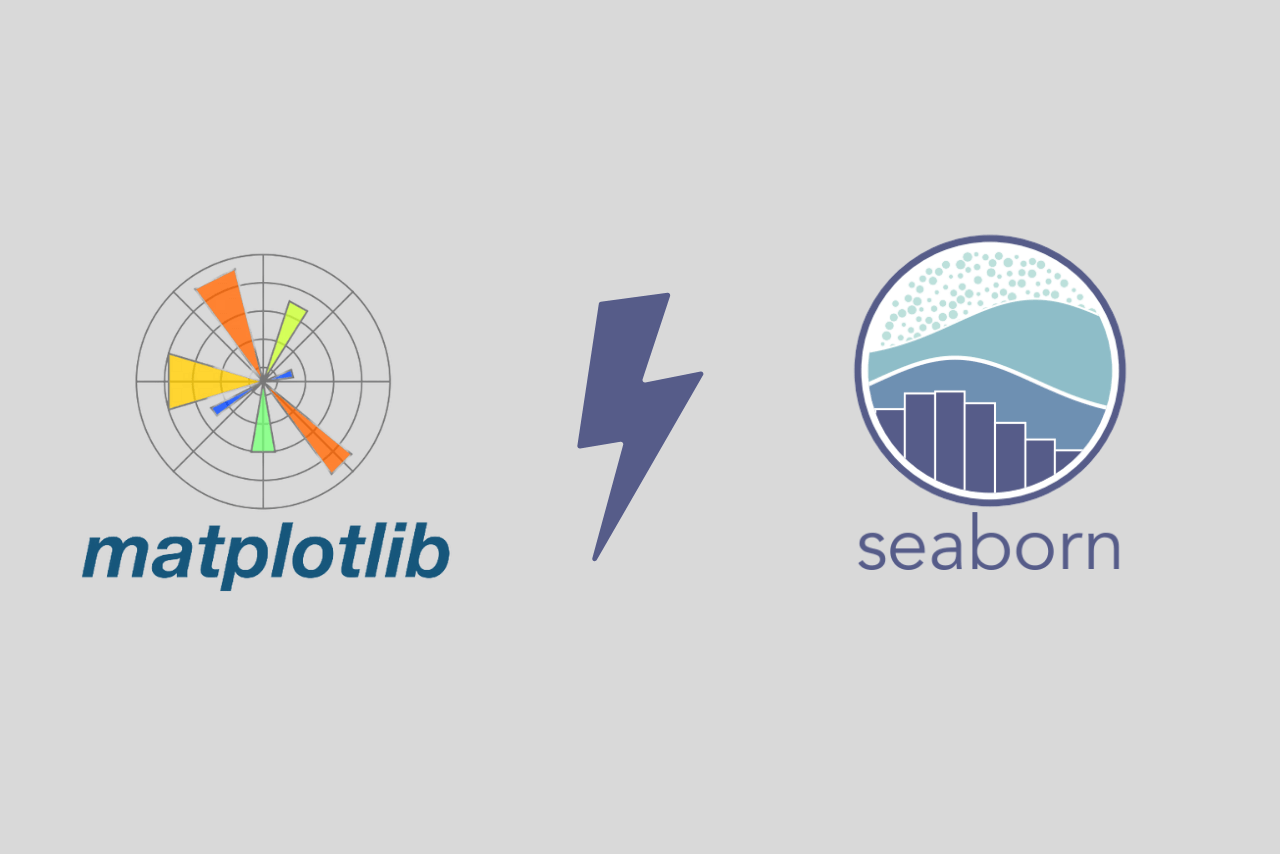
Next Steps and Resources
Congratulations! You've made significant progress in your Python journey. In this section, we'll guide you on what to explore next and provide resources to continue your Python learning adventure.
Advanced Topics
Python offers a wide range of advanced topics to explore, depending on your interests. You can dive into multi-threading, which allows your program to perform multiple tasks simultaneously. If web development is your passion, consider learning Flask or Django. For those interested in machine learning, libraries like TensorFlow and scikit-learn offer exciting opportunities.
Online Resources
To continue your Python education, we recommend exploring online resources such as websites, courses, and forums. Websites like Python.org and Python Package Index (PyPI) are essential sources for documentation and packages. Online courses on platforms like Coursera, edX, and Udemy offer in-depth Python tutorials. Additionally, communities like Stack Overflow and Reddit's r/learnpython can be valuable for seeking help and sharing knowledge with other Python enthusiasts.
Conclusion
In conclusion, learning Python is an exciting and rewarding journey. We've covered the fundamentals, data structures, object-oriented programming, error handling, and essential libraries. The key to mastering Python is practice and experimentation. As you continue to work on your projects and explore advanced topics, you'll become a proficient Python developer. Keep your curiosity alive and stay engaged with the ever-growing Python community, and you'll find yourself accomplishing great things with this versatile language. Happy coding!

FAQs About Learning Python
What is Python, and why should I learn it?
Python is a high-level, versatile programming language known for its simplicity and readability. Learning Python opens up opportunities in web development, data analysis, automation, and more. Its large and active community ensures constant growth and support.
Do I need any prior programming experience to learn Python?
No, Python is an excellent choice for beginners. Its straightforward syntax and extensive libraries make it accessible even if you have no prior programming experience.
How can I install Python on my computer?
Installing Python is easy. You can download the latest version from the official Python website (python.org) and follow the installation instructions for your specific operating system.
What is the best way to start learning Python as a beginner?
Start by learning the basics of Python syntax, variables, and data types. Then, progress to control structures like if statements and loops. Practicing coding exercises and projects is crucial for reinforcing your knowledge.
Are there any recommended coding environments or IDEs for Python?
Python can be written in any text editor, but many developers prefer using Integrated Development Environments (IDEs) like PyCharm, Visual Studio Code, or Jupyter Notebook for a more streamlined coding experience.